When you’re working with Vue2, one of the most common frustrations is understanding how v-if
, v-for
, and their “vue2 v-if权重 vfor” (priority or weight) interact.
This topic has caused countless developers to scratch their heads.
How does Vue decide what to evaluate first? Why do things break when these directives overlap? Let’s break it all down in plain language.
What Is “Vue2 v-if权重 vfor” All About?
In Vue2, v-if
and v-for
are directives that control what gets rendered on the page.
v-for
loops through a list of items and renders them.v-if
conditionally renders content based on a condition.
The tricky part? When you use them together, their “vue2 v-if权重 vfor” determines which one takes priority. And that can affect how your app behaves.
Here’s the key rule: v-for
always runs first.
Let’s unpack that with an example.
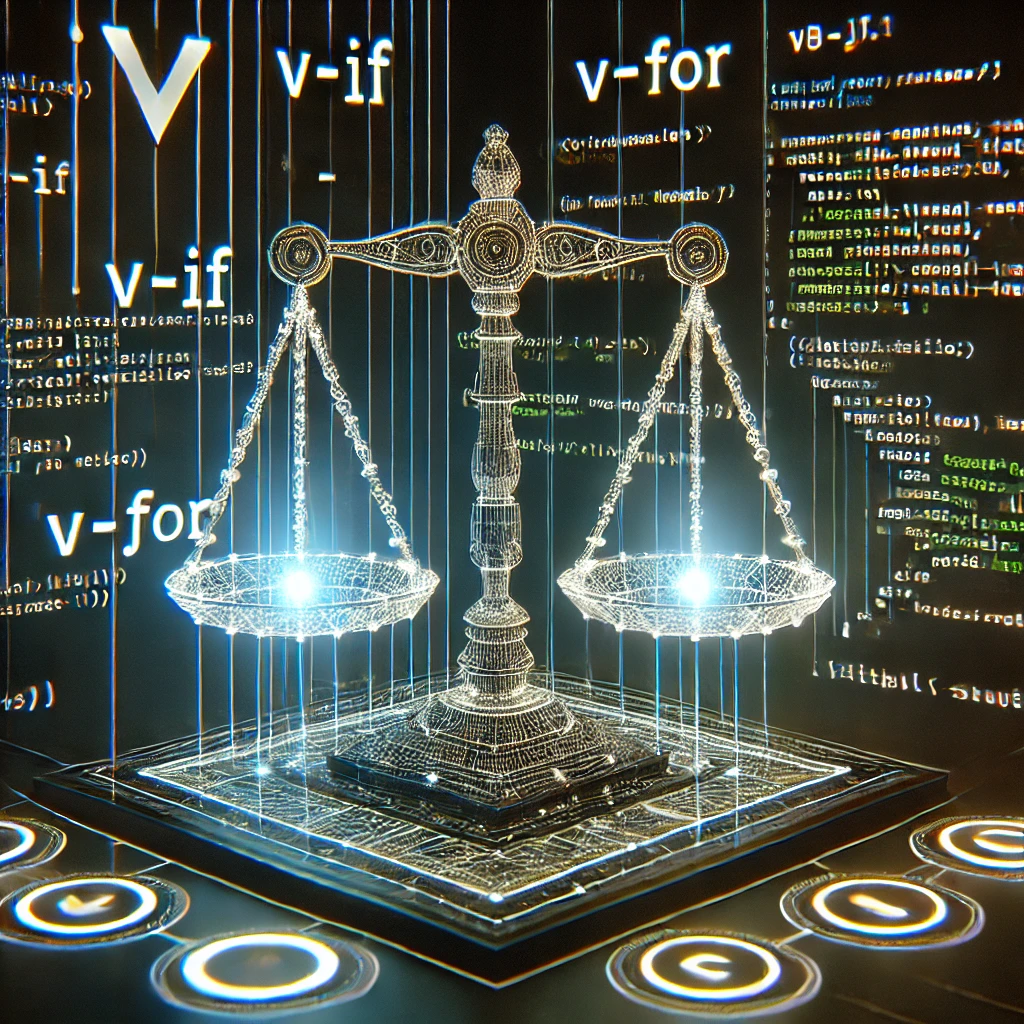
A Real-Life Example: Mixing v-for and v-if
Imagine you’re building a to-do list app.
You have an array of tasks, and you only want to show tasks that are marked as “important.”
vueCopy code<li v-for="task in tasks" v-if="task.important">
{{ task.name }}
</li>
At first glance, this seems fine. But Vue throws a warning.
Why?
Because v-for
runs before v-if
. Vue loops through every task, then checks the condition. That’s a performance hit, especially if your list has 1,000+ tasks.
The Right Way to Combine vue2 v-if权重 vfor
Vue recommends moving the v-if
condition outside the loop. This avoids unnecessary rendering.
Here’s how:
vueCopy code<li v-for="task in filteredTasks">
{{ task.name }}
</li>
And define filteredTasks
in your computed properties:
javascriptCopy codecomputed: {
filteredTasks() {
return this.tasks.filter(task => task.important);
}
}
This way, Vue filters the list first, then loops through the smaller, filtered array.
Why Does Vue Handle It This Way?
Vue prioritizes performance.
If v-if
ran before v-for
, Vue would need to evaluate the condition for every loop iteration, even if the loop has 1,000+ items.
By letting v-for
handle the heavy lifting first, Vue minimizes unnecessary work.
FAQs About Vue2 v-if权重 vfor
Q: Can I ever use vue2 v-if权重 vfor for
together?
A: Yes, but it’s not recommended for large lists. Stick to filtering your data before passing it to v-for
.
Q: What happens if I ignore Vue’s warnings?
A: Your app might work fine with small datasets. But as your data grows, performance will take a big hit.
Q: Is this behavior the same in Vue3?
A: Mostly, yes. But Vue3 has better reactivity and optimized rendering, making it less of a concern.
Quick Tips for Using vue2 v-if权重 vfor
- Filter your data upfront.
Use computed properties or methods to filter lists before rendering them. - Avoid combining
v-if
andv-for
directly.
It works, but it’s bad for performance and can cause unnecessary bugs. - Understand Vue’s rendering priority.
Always remember:v-for
first, thenv-if
. - Test with larger datasets.
What works on small arrays might break or slow down with thousands of items. - Use the Vue Devtools.
They can help you debug rendering issues quickly.
Real-World Applications of This Knowledge
Think of a movie app showing a list of films. If users want to see “Top Rated” movies, you don’t filter them inside the v-for
loop.
Instead, use a computed property to filter the list, then render only the top-rated ones.
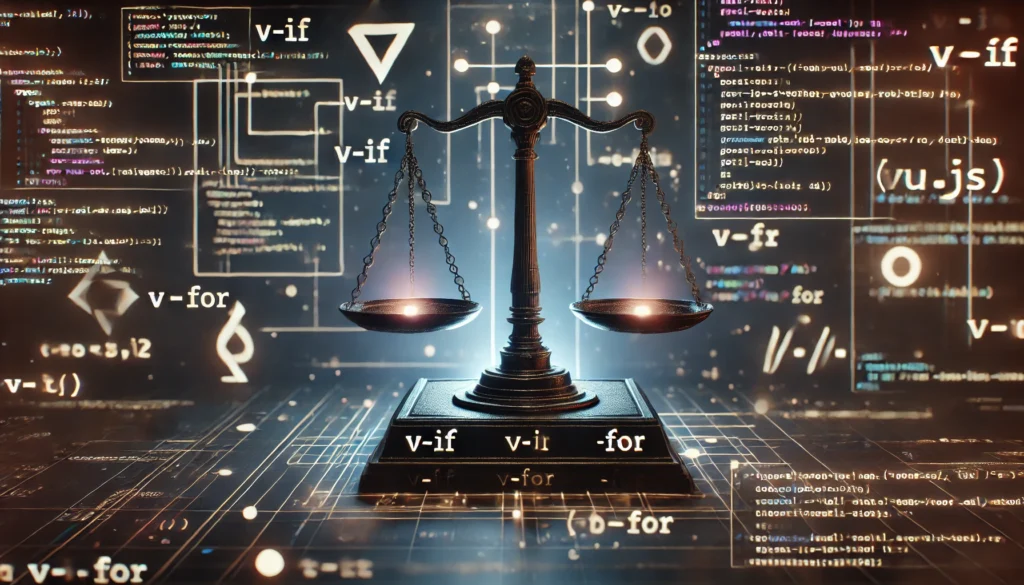
Where to Learn More About Vue2 v-if权重 vfor
If this concept still feels tricky, check out these resources for more examples:
- Vue2 Documentation on Directives
- Stack Overflow Discussions on Vue2
- Vue Mastery’s Deep Dive into v-if and v-for
Wrapping Up
“Vue2 v-if权重 vfor” might seem confusing at first, but understanding their priorities will save you hours of debugging.
By filtering data before looping and keeping performance in mind, you’ll write cleaner, faster, and more maintainable Vue apps.
Remember: Vue2 v-if权重 vfor isn’t a bug; it’s a feature.
Master it, and you’ll handle complex UIs like a pro.